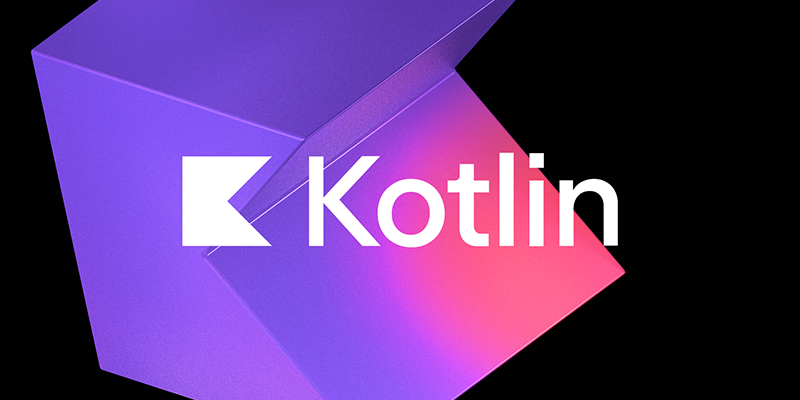
Kotlin Variables
In any programming language variables are used to store data. The type of the variable decides what kind of data it will store.
Variables in Kotlin are of two types :-
1. Immutable variable (declared with val)
2. Mutable variable (declared with var)
Immutable Variable
Immutable variables are the variables which are declared using the val keyword. These type of variables can only be assigned once. If we try to reassign immutable variables an error will be thrown by the compiler
// immutable variable declaration example
val name = "Amit"
name = "Ankit" // This line thrown compile time error
Mutable variable
Mutable variable is a variable which are declared using var keyword. the value of these type of variables can be changed during runtime.
// mutable variable declaration example
var name = "Amit"
name = "Ankit" // no compile time error
NOTE :
What is the diff in val and const in Kotlin ?
val : The value of val can be assigned at runtime. it is not necessary to assign a value at compile time.
const : The value of const should be known at compile time else the compiler will throw an error.
Variable Naming convention
The naming convention of variable should follow lowerCamelCase.
val firstName = "Amit"
val lastName = "Gupta"
Comments
Post a Comment