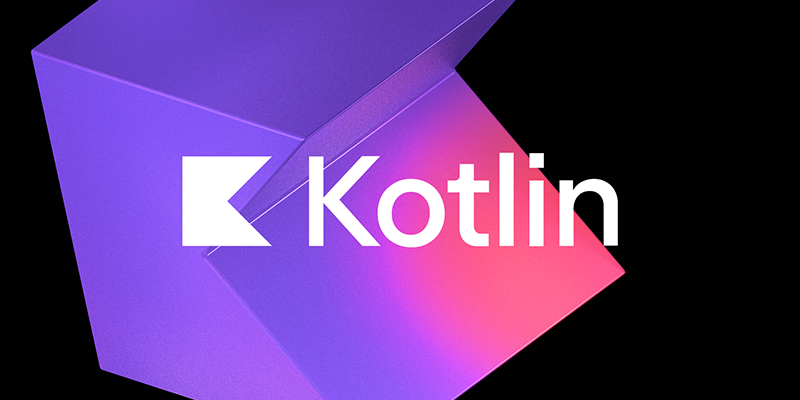
1. Lambda Expressions : Lambda Expressions are short block of code that takes in some parameters and returns a value. Lambda expressions do not have a name and can be passed as functions parameters.
var expression = { a : Int, b : Int ->
a + b
}
return type will be inferred automatically from the return statement. Below is the example of passing lambda function as function parameter
fun main(args : Array<String>) {
processData(1, 1, { a: Int, b : Int -> a + b})
}
fun processData(a : Int, b : Int, c : (Int, Int) -> Int) {
println(c(a, b))
}
2. Anonymous functions : Anonymous functions are functions without name.
It is same as a regular function but it is without name.
var res = fun(num1 : Int, num2 : Int) -> Int {
return num1 + num2
}
var res = fun(num1 : Int, num2 : Int) -> Int = num1 + num2
Comments
Post a Comment